When to use using and when to use try catch to do the cleaning
- We have two ways to clean up utilized resources in C# either we can use try..catch..finally statements series or we can use the using statement in C#
- The thing is that resources should be released and cleanup must be performed either before exitting the using block or just entering up the finally block.
- Use using Statement in C# to release up the resources when the creating type implements the IDisposable interface.
- Use try..catch..finally when we need to perform cleanup but not necessarily employ the Dispose Method.
Installing Intel X3100 Graphics Driver on Toshiba Sattellite M200 Laptops having WS2003 as Operating Sytem
Those of you who are having trouble in installing X3100 Graphics Driver on Toshiba Satellite M200 and similar laptops in that series, the problem is that the driver that toshiba people have on their site is old version and it works only on Windows XPSP2 and later OS,it does not work on Windows Server 2003 OS. So the solution is just download the Graphics Driver for Windows XP for Dell Inspiron 1525. These dell people have latest driver and it install very smoothly on Windows Server 2003. If this also is not working for you than you can add setup.exe in the application verifier and do the proceeding as given in this post. https://www.smallworkarounds.com/index.php/2008/09/17/installing-photoshop-cs3-on-windows/
Anonymous Types in C#
- Anonymous types in C# are a new concept which shipped with .net framework 3.5
- Anonymous types are nothing but it simply states that we dont have to define a type and that type is defined automatically by C# analysing the right hand side.
- Anonymous Types are very important in the LINQ word.
- Keyword var is used to declare an anonymous type.
- They are immutable and thus dont require any property getter or setter methods
- They must always be initialized so that the compiler can build the type defination and bind the anonymous type with that , and it is only possible only when we have any thing in the right hand side of an anonymous type thus anonymous types should always be initialized.
//1.Shows Simple anonymous varible declarations and iterating using for loop
1: var fabonici = new int[]{1,1,2,3,5,8,13,21};
2: for( var i = 0; i<fabonici.Length; i++)
3: Console.WriteLine(fabonici[i]);
4:
//2.Show Simple anonymous variable declarations and iterating using foreach loop
1: var fabonici = new int[]{1,1,2,3,5,8,13,21};
2: foreach (var fibo in fabonici)
3: Console.WriteLine(fibo);
//3.Linq query used as iterand in foreach statement.
1: var fabonici = new int[]{1,1,2,3,5,8,13,21};
2: foreach (var fibo in
3: from f in fabonici
4: where f%3 == 0
5: select f)
6: Console.WriteLine(fibo);
Custom DAL having functions which recieve StoredProcedure Name and SortedList having key/value pairs as parameters to reduce code duplicacy by minimizing open() and close() connection.
Mostly whenever we code an enterprise project we should follow the n-tier model and divide our project into various tiers. Lets continue from the UI,so first tier is our UI ,from the UI we should we should make use of our Model layer objects which simply contains classes which map our requirements for the projects. Now from UI,we should create objects of Model Classes and pass these model classes to the Service class.This service class is nothing but it contains all the static functions which we require in our projects.Most of the application's service logic is applied in these classes, or we can say that these classes serve the Model Objects behaviour. Next comes the most important layer called Utility or some people might Directly call it DAL layer. Anyways the function of this layer is to interact with the Service Layer on one hand and on the other hand fetch and persist result to the database either using ad-hoc queries or using the stored procedure logic.Given below is the representation of this n-tier project arichitecture
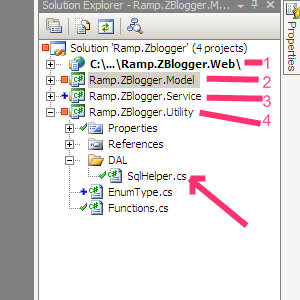
In this article we will mainly concentrate on the DAL part or the last layer or tier in our architecture.In our model we are not using ad-hoc queries but only stored procedures.So we have developed a SqlHelper file which will recieve only the names of stored procedure and a sorted list having a key value pair of parameters which we will pass to the stored procedure. This layer will also handle every opening and closing of the connection to the database.We generally return a reader from the database to any point in our code but here we don't want to open and close connection in the application logic so we are converting the reader fetched from the database to the dataset and then finally returning the dataset in the application. There are various functions used in order to achieve this logic i will describe here a few important ones.You can download the whole file which is attached at the end of this article.
1: public static void DBExecuteNonQuery(string storedProcedure, SqlCommand command)
2: {
3: using (var connection = new SqlConnection(ConnectionString))
4: {
5: connection.Open();
6: command.CommandText = storedProcedure;
7: command.Connection = connection;
8: command.CommandType = CommandType.StoredProcedure;
9: command.ExecuteNonQuery();
10: }
11: }
We are not using above function directly from the application service layers but it is being called from inside another sqlhelper function called "ConvertToSqlCommandForNonQuery" although it can be directly used but we want to follow our key,value transfer from the application thus we follow this pattern.So below given is our ConvertToSqlCommandForNonQuery function which takes stored procedure name and sortedlist as parameters,then it calls the DBExecuteNonQuery which we have seen just above after making a command out of the recieved SoretedList.
In this function we are also using a ConvertToSqlDBType function, it is used because the value we recieve as object and we now have to map the objects C# type to Sql Server Database type.So we have another function called ConvertToSqlDBType to achieve this.We will talk about it later.
1: public static void ConvertToSqlCommandForNonQuery(string storedProcedure, SortedList<string, object> values)
2: {
3: var command = new SqlCommand();
4: foreach (var value in values)
5: {
6: Type type = value.Value.GetType();
7: SqlDbType sqlDBType = ConverToSqlDBType(type);
8: command.Parameters.Add(value.Key, sqlDBType).Value = value.Value;
9: }
10: DBExecuteNonQuery(storedProcedure, command);
11: }
1: public static void SaveCategory(Category category)
2: {
3: var parameterList = new SortedList<string, object>();
4: parameterList.Add("categoryName", category.CategoryName);
5: SqlHelper.ConvertToSqlCommandForNonQuery("usp_SaveCategory", parameterList);
6: }
1: public static Int32 DBExecuteScalar(string storedProcedure, SqlCommand command)
2: {
3: Int32 value = 0;
4: using (var connection = new SqlConnection(ConnectionString))
5: {
6: connection.Open();
7: command.CommandText = storedProcedure;
8: command.Connection = connection;
9: command.CommandType = CommandType.StoredProcedure;
10: value = (Int32) command.ExecuteScalar();
11: }
12: return value;
13: }
1: public static Int32 DBExecuteScalar(string storedProcedure, SortedList<string, object> values)
2: {
3: var command = new SqlCommand();
4: foreach (var value in values)
5: {
6: Type type = value.Value.GetType();
7: SqlDbType sqlDBType = ConverToSqlDBType(type);
8: command.Parameters.Add(value.Key, sqlDBType).Value = value.Value;
9: }
10: int retValue = DBExecuteScalar(storedProcedure, command);
11: return retValue;
12: }
Third set of functions are the main functions which first convert the datareader returned from the stored procedure to the dataset and then return this dataset to the application.We can very well return the datareader to the application and then iterate till the end of the reader but we are not doing here because we dont want to carry the opened connection to the application or simply we want to limit the connection flow only to the DAL layer.
1: public static DataSet DBExecuteReader(string storedProcedure)
2: {
3: SqlDataReader reader = null;
4: var command = new SqlCommand();
5: using (var connection = new SqlConnection(ConnectionString))
6: {
7: connection.Open();
8: command.CommandText = storedProcedure;
9: command.Connection = connection;
10: command.CommandType = CommandType.StoredProcedure;
11: reader = command.ExecuteReader();
12:
13: var dataSet = new DataSet();
14: do
15: {
16:
17: DataTable schemaTable = reader.GetSchemaTable();
18: var dataTable = new DataTable();
19:
20: if (schemaTable != null)
21: {
22: for (int i = 0; i < schemaTable.Rows.Count; i++)
23: {
24: DataRow dataRow = schemaTable.Rows[i];
25: var columnName = (string) dataRow["ColumnName"];
26: var column = new DataColumn(columnName, (Type) dataRow["DataType"]);
27: dataTable.Columns.Add(column);
28: }
29:
30: dataSet.Tables.Add(dataTable);
31: while (reader.Read())
32: {
33: DataRow dataRow = dataTable.NewRow();
34:
35: for (int i = 0; i < reader.FieldCount; i++)
36: dataRow[i] = reader.GetValue(i);
37:
38: dataTable.Rows.Add(dataRow);
39: }
40: }
41: else
42: {
43:
44:
45: var column = new DataColumn("RowsAffected");
46: dataTable.Columns.Add(column);
47: dataSet.Tables.Add(dataTable);
48: DataRow dataRow = dataTable.NewRow();
49: dataRow[0] = reader.RecordsAffected;
50: dataTable.Rows.Add(dataRow);
51: }
52: } while (reader.NextResult());
53: return dataSet;
54: }
55: }
1: public static DataSet DBExecuteReader(string storedProcedure, SqlCommand command)
2: {
3: SqlDataReader reader = null;
4: using (var connection = new SqlConnection(ConnectionString))
5: {
6: connection.Open();
7: command.CommandText = storedProcedure;
8: command.Connection = connection;
9: command.CommandType = CommandType.StoredProcedure;
10: reader = command.ExecuteReader();
11:
12:
13: var dataSet = new DataSet();
14: do
15: {
16:
17: DataTable schemaTable = reader.GetSchemaTable();
18: var dataTable = new DataTable();
19:
20: if (schemaTable != null)
21: {
22:
23: for (int i = 0; i < schemaTable.Rows.Count; i++)
24: {
25: DataRow dataRow = schemaTable.Rows[i];
26:
27: var columnName = (string) dataRow["ColumnName"];
28:
29: var column = new DataColumn(columnName, (Type) dataRow["DataType"]);
30: dataTable.Columns.Add(column);
31: }
32:
33: dataSet.Tables.Add(dataTable);
34:
35:
36: while (reader.Read())
37: {
38: DataRow dataRow = dataTable.NewRow();
39:
40: for (int i = 0; i < reader.FieldCount; i++)
41: dataRow[i] = reader.GetValue(i);
42:
43: dataTable.Rows.Add(dataRow);
44: }
45: }
46: else
47: {
48:
49: var column = new DataColumn("RowsAffected");
50: dataTable.Columns.Add(column);
51: dataSet.Tables.Add(dataTable);
52: DataRow dataRow = dataTable.NewRow();
53: dataRow[0] = reader.RecordsAffected;
54: dataTable.Rows.Add(dataRow);
55: }
56: } while (reader.NextResult());
57: return dataSet;
58: }
59: }
1: public static DataSet ConvertToSqlCommand(string storedProcedure, SortedList<string, object> values)
2: {
3: var command = new SqlCommand();
4: foreach (var value in values)
5: {
6: Type type = value.Value.GetType();
7: SqlDbType sqlDBType = ConverToSqlDBType(type);
8:
9: command.Parameters.Add(value.Key, sqlDBType).Value = value.Value;
10:
11: }
12: DataSet dataSet = DBExecuteReader(storedProcedure, command);
13: return dataSet;
14: }
Given below is the example of the "ConvertToSqlCommand" function.Here we are populating all the categories of a particular user.
1: public static List<Category> PopulateUserCategory(int userID)
2: {
3: var categoryList = new List<Category>();
4: var parameterList = new SortedList<string, object>();
5: parameterList.Add("@userID", userID);
6: DataSet dataSet = SqlHelper.ConvertToSqlCommand("usp_GetAllCategoriesByUserID", parameterList);
7: DataTable dataTable = dataSet.Tables[0];
8: foreach (DataRow dataRow in dataTable.Rows)
9: {
10: var category = new Category();
11: category.CategoryID = Convert.ToInt32(dataRow["CategoryID"]);
12: category.CategoryName = Convert.ToString(dataRow["CategoryName"]);
13: categoryList.Add(category);
14: }
15: return categoryList;
16: }
This is the "ConvertToSqlDBType" function which we use to convert C# types to SqlDBTypes.Given below are some common type conversions you can very well enhance this list and do whatever you feel like.
1: public static SqlDbType ConverToSqlDBType(Type systemType)
2: {
3:
4: switch (systemType.Name)
5: {
6: case "Int32":
7: return SqlDbType.Int;
8: case "String":
9: return SqlDbType.NVarChar;
10: case "DateTime":
11: return SqlDbType.DateTime;
12: case "Boolean":
13: return SqlDbType.Bit;
14: default:
15: return SqlDbType.VarChar;
16: }
17: }
Conclusion
rpc_relay
canvas
<html> <head>
<style type="text/css">
/*
These styles are customizable.
Provide .canvas-gadget (the div that holds the canvas mode gadget)
at least 500px width so that the gadget has sufficient screen real estate
*/
body {
margin: 0;
font-family:arial, sans-serif;
text-align:center;
}
.container {
width:652px;
margin:0 auto;
text-align:left;
}
.fc-sign-in-header {
text-align:left;
font-size: 13px;
padding:3px 10px;
border-bottom:1px solid #000000;
}
.signin {
text-align:left;
float:right;
font-size: 13px;
height: 32px;
}
.go-back {
text-align:left;
margin:5px auto 15px auto;
}
.go-back a, .go-back a:visited {
font-weight:bold;
}
.canvas-gadget {
text-align:left;
width:650px; /* ALLOW AT LEAST 500px WIDTH*/
margin:10px auto 10px auto;
border:1px solid #cccccc;
}
.site-header {
margin-top: 10px;
}
.section-title {
font-size: 2em;
}
.clear {
clear:both;
font-size:1px;
height:1px;
line-height:0;
margin:0;
padding:0;
}
</style>
<script type="text/javascript" src="
How to make a function accepting variable arguments of same type in C#
Here is a simple way by which we can declare a function in C# which accepts variable arguments of a particular type. We have a keyword params in C# which we can use in the parameters in a function.
public class Util { public static List<int> AddIntegersToList(params int[] integerList) { List<int> numbers = new List<int>(); foreach (int item in integerList) { numbers.Add(item); } return numbers; } }
Now this will accept an array of integers and thus the no of integers which can be passed here is not fixed and hence it fullfills our requirement of a function which can receive variable no of arguments of a single type.
Now for the above function we can use
List<int> listOfIntegers = Util.AddIntegersToList(2, 8, 9, 56, 77);
We can pass here any no of integers.
window.google_render_ad();
Boxing And Unboxing in C#
1. Boxing is when we convert a value type into reference type,when we are boxing we are creating reference variables which point to a new copy on the heap. 2. Unboxing is when we convert a reference type to a value type. 3. Best example of boxing and unboxing can be while we store integer variable which is a value type in a ArrayList which is a reference type. 4. And when we retrieve the value stored in ArrayList to an integer then we change a reference type to value type ie we do unboxing.
ArrayList myArrayList = new ArrayList(); Int n = 7; myArrayList.Add(n);//Boxing of n n = (int)myArrayList[0];//Unboxing of myArrayList[0]
Two uses of using keyword in C#
There are two uses of using keyword in C# i)Using as Directive in C# can be used for importing namespaces to your classes. ii)Another most useful advantage is it can be used in codeblocks what is does is it calls the Dispose() method automatically to release the resources and also automatically implements the try catch blocks for you.Here it is called a using statement.So the advantage is that we dont have to explicitly write a try-catch block and our code looks tidy.
Installing Photoshop CS3 on Windows Server 2003 Systems
Hi yesterday i was trying to install Photoshop CS3 on a system having Windows Server 2003 installed. But it gives me an error saying that you need a OS equivalent to Windows XP SP2 or greater or Windows Vista. So i found a workaroud which i would like to share with you. Just download a utility from Microsoft called Application Verifier its a small but very benificial utility.Link is http://www.microsoft.com/downloads/details.aspx?FamilyID=C4A25AB9-649D-4A1B-B4A7-C9D8B095DF18&displaylang=en 1.Run the Application Verifier and open the setup.exe file for photoshop cs3 and go to the compatibility tree view column. 2.Go to HighVersionLie and right click it to get properties. 3.Now suppose if you want to any program assume that you are using Windows XP SP2 then enter like below figure in the boxes that follow
4.Click OK And then Save 5.It will then ask you for the debugger to be attached do not attach any debugger and click save . 6.Now if you try installing it will be installed with no problems.
- 1
- 2